35.4 Glade Reference
35.4.1 Writing Your Code
35.4.1.1 Finding Your Widget
If you use glade to generate source code for your interface (rather
than using ) you should make use of the
lookup_widget()
function that glade defines for you (in
support.c
) to access your widgets. You pass this function a
pointer to any widget in a window and the name of the widget that you
want to get (where the name is a string and is the same as the
Name in the Properties dialog for the widget). The
function will return a pointer to the widget whose name matches the
string you supply.
The lookup_widget()
function relies on you giving a
pointer to any other widget in the same tree (perhaps a pointer to the
root of the widget hierarchy for that particular application window or
dialog). Usually in a signal handler (the callbacks that you write in
callbacks.c
) you can use the first argument to the signal
handler as the first parameter to lookup_widget()
. For
example you may have a button in you window called button1
and when it is clicked you may want to access some text entry field
that has the name ``entry1
.’’ In callbacks.c
you may have a callback:
void
on_button1_clicked (GtkButton *button,
gpointer user_data)
{
GtkWidget *entry1;
entry1 = lookup_widget (GTK_WIDGET (button), "entry1");
...
}
Internally glade uses gtk_object_set_data()
for
storing pointers to all the widgets in a window using the names set in
the property editor as the key. Then, inside
lookup_widget()
, gtk_object_get_data()
is
used to retrieve the pointer indexed by this key. These two functions
are also available to the developer for their own purposes.
35.4.1.2 Using the GTKExtra Libraries
To make use of these libraries you will need to add the appropriate
libraries to your src/Makefile.in
and make appropriate
modifications to autogen.sh
and configure.in
. Or
perhaps you ONLY need to add to the appropriate LIBS line in
src/Makefile.am
. This seems more likely.
35.4.1.3 Global and Local Pointers
An eternal issue in GUI development is how to get hold of the pointer to the individual widgets when you need them. Should they be accessed from global pointers that then restrict you to a single instance (or a known number of instances) of the widgets a priori, or is there a better mechanism?
35.4.1.3.1 Global Top Level Widgets
One solution is to have the top level widgets global. This then allows
all child widgets to be accessed using lookup_widget()
provided by glade.
35.4.2 Options for the Command Line
Normally you construct your GUI interactively with glade and then choose to build the C code for the interface with the Build button. It is sometime inconvenient to fire up glade just to build the C source code from the glade XML file. Instead you can do the conversion from the command line with:
If the command line option is not supplied, but a gladefile
is supplied, then glade will start up and load the specified
project.
35.4.4 Project Options
The Project Options dialog allows you to tune many aspects of the project you are developing with glade. The types of options fall under three tabs: General, C Options, and LibGlade Options.
35.4.4.1 General
The General options cover the project location, project names, programming language, and if to support GNOME.
35.4.4.1.1 Basic Options
The Project Directory lists the directory in which the saved project file will be stored. This is also where the built source code will be written. Use the Browse button to identify a directory using a File Selection Dialog (which allows you to also create directories if you wish). As you change the the Project Name, Program Name, and Project File values will also change, unless you have already changed these other fields separately to give them a different name.
When you save or build a project the directories will be silently created as needed.
The Project Name is the name of your project. This will be used as the title of the main application window you create (the GNOME Application Window). The name can have spaces and other characters. As you change the name of the project the , and Project File fields also change to reflect your project name (with spaces and other non-alphanumeric characters converted to dashes).
The Program Name is the name by which the final executable program will be known. This is used by glade to name the output executable file in the Makefile it generates. Once again, as you change this field the following field (Project File) automatically changes to be the same. The previous fields do not change.
The Project File is then the file located in the
Project Directory in which the interface is saved. This file
has the extension .glade
and is a gzip’ed XML document that
records all of the project information. See
Section 35.5.3 for details.
35.4.4.1.2 Subdirectories
The Subdirectories fields indicate where glade should place source code files it generates (Source Directory) and where glade should place any pixmaps (graphics) used in the project (Pixmaps Directory). These subdirectories are located in the Project Directory and are silently created by glade when it builds the project.
35.4.4.1.3 Language
A choice of languages is available under Language. Only one can be chosen at a time. The choice tells glade what to do when the Build button or menu is chosen. See Section 35.4.3.1.4 for details. The choices are C, C++, Ada95, Perl, and Eiffel. Note that this is not relevant when using libglade as the conversion is effectively performed at run time.
Your donation will support ongoing availability and give you access to the PDF version of this book. Desktop Survival Guides include Data Science, GNU/Linux, and MLHub. Books available on Amazon include Data Mining with Rattle and Essentials of Data Science. Popular open source software includes rattle, wajig, and mlhub. Hosted by Togaware, a pioneer of free and open source software since 1984. Copyright © 1995-2022 Graham.Williams@togaware.com Creative Commons Attribution-ShareAlike 4.0
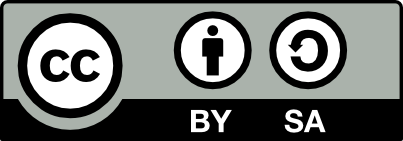